前言
今天学习了阿里云的视频点播VoD,记录一下搭建与阿里云VoD的对接服务
本篇同样是阿里云的云服务,有些地方类似的操作(比如开通服务流程、创建子账号、权限分组等)就不重复详细记录了直接一笔带过。类似的操作OSS服务搭建中有详细步骤,可以作为参考
什么是视频点播VoD
摘自阿里云视频点播VoD文档:
https://www.aliyun.com/product/vod
视频点播(ApsaraVideo VoD,简称VoD)是集视频采集、编辑、上传、媒体资源管理、自动化转码处理(窄带高清TM)、视频审核分析、分发加速于一体的一站式音视频点播解决方案。
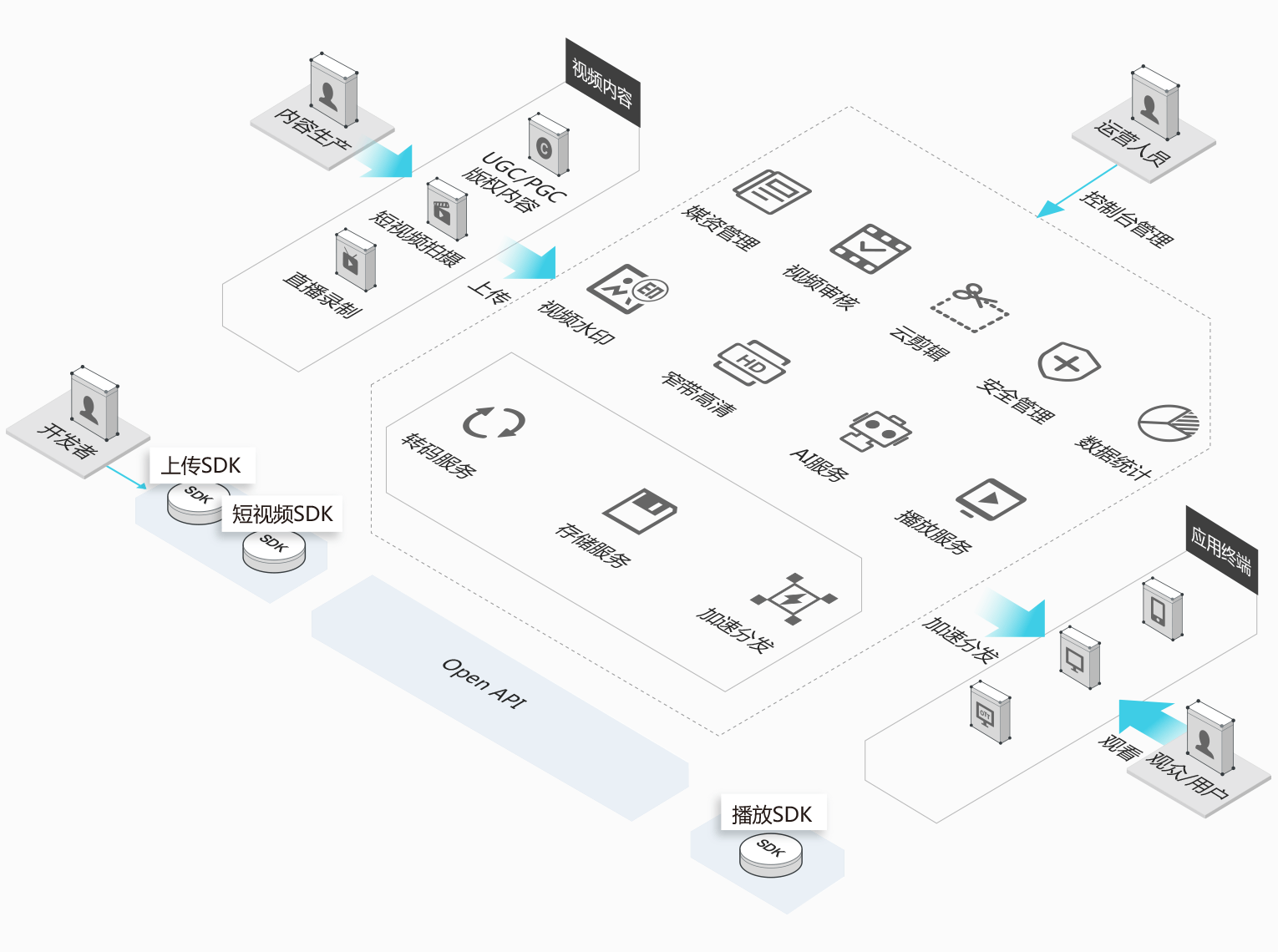
计费规则
https://www.aliyun.com/price/product?spm=5176.8413026.1397775..1a0b11cfE7n8nG&aly_as=sXiM5Y4IA#/vod/detail
学习的话充个几块钱即可
开通VoD
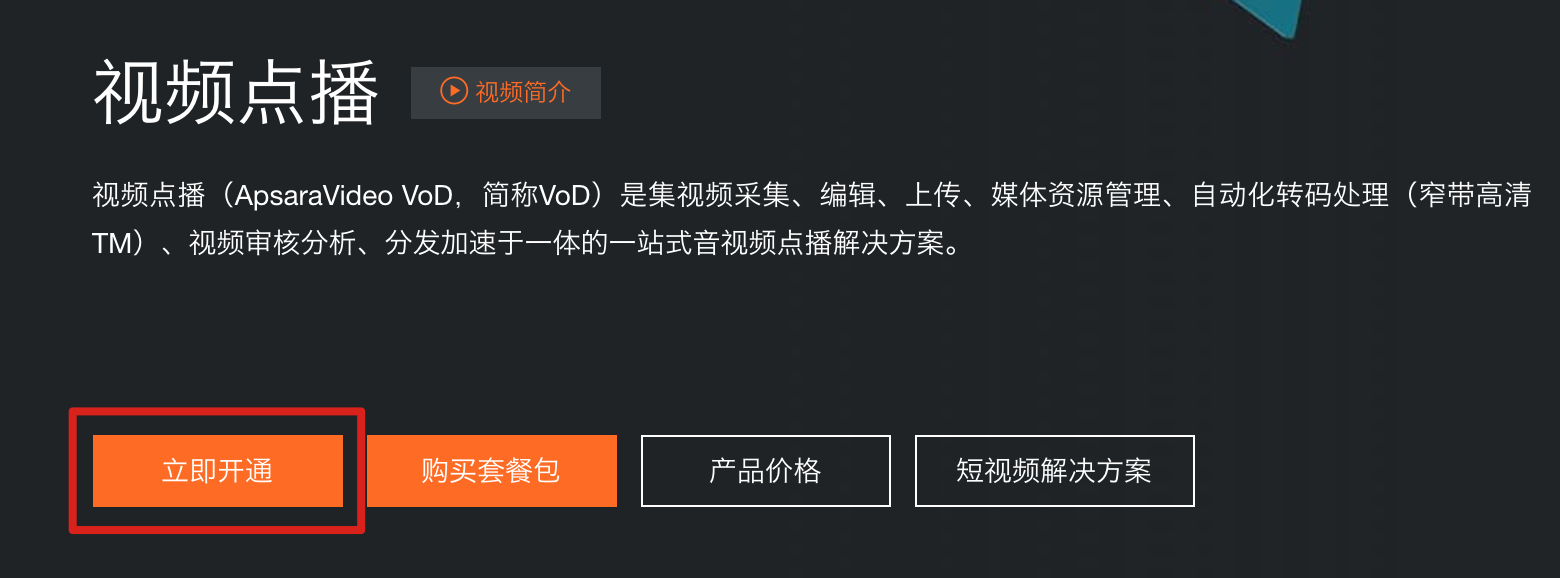
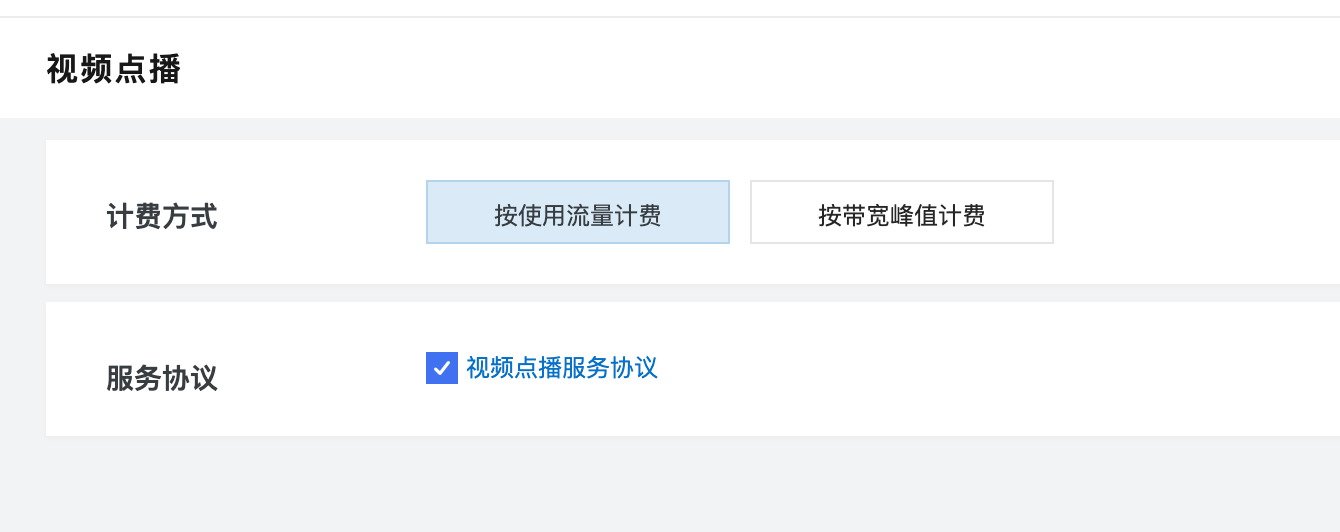
VoD管理后台
上传一个自己的视频
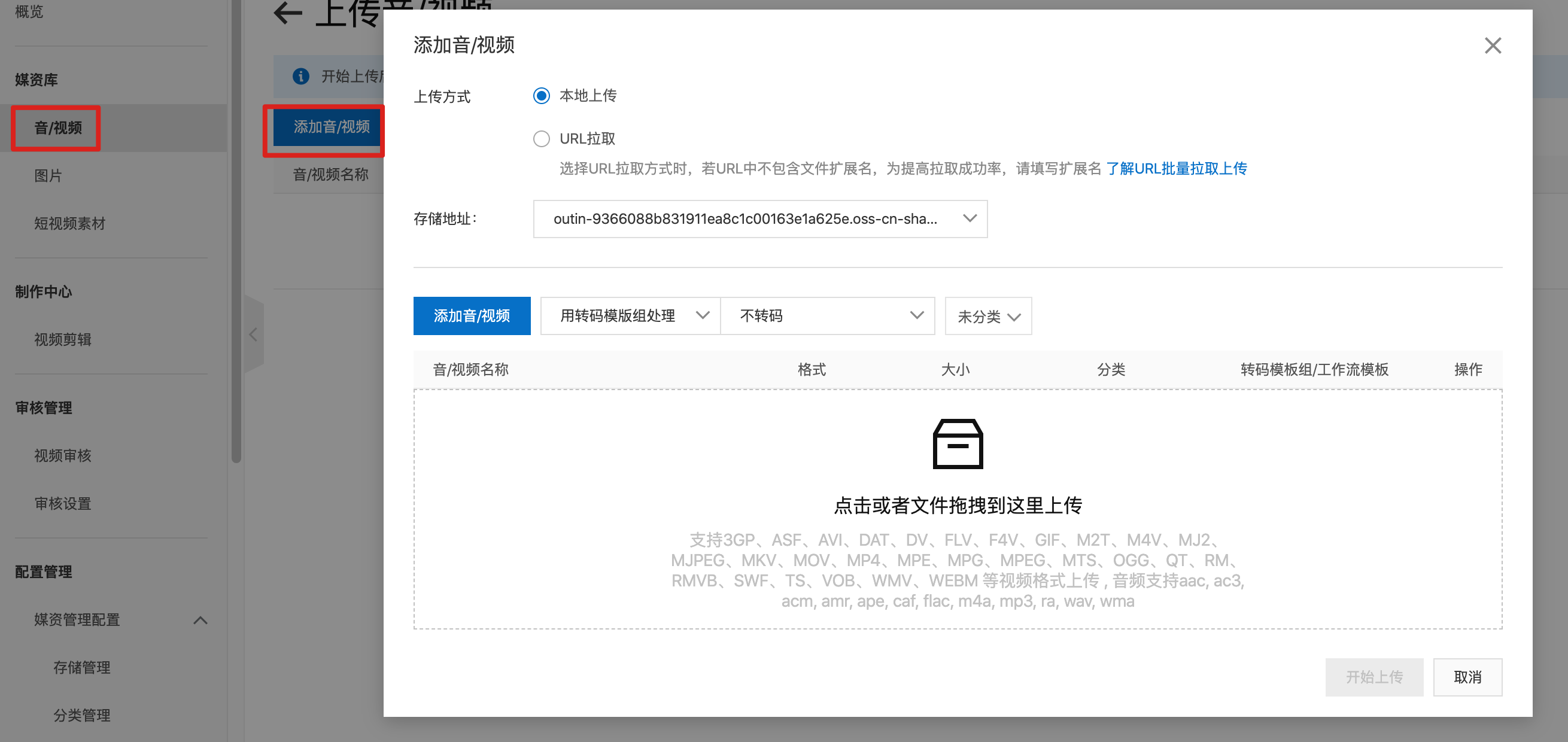
转码
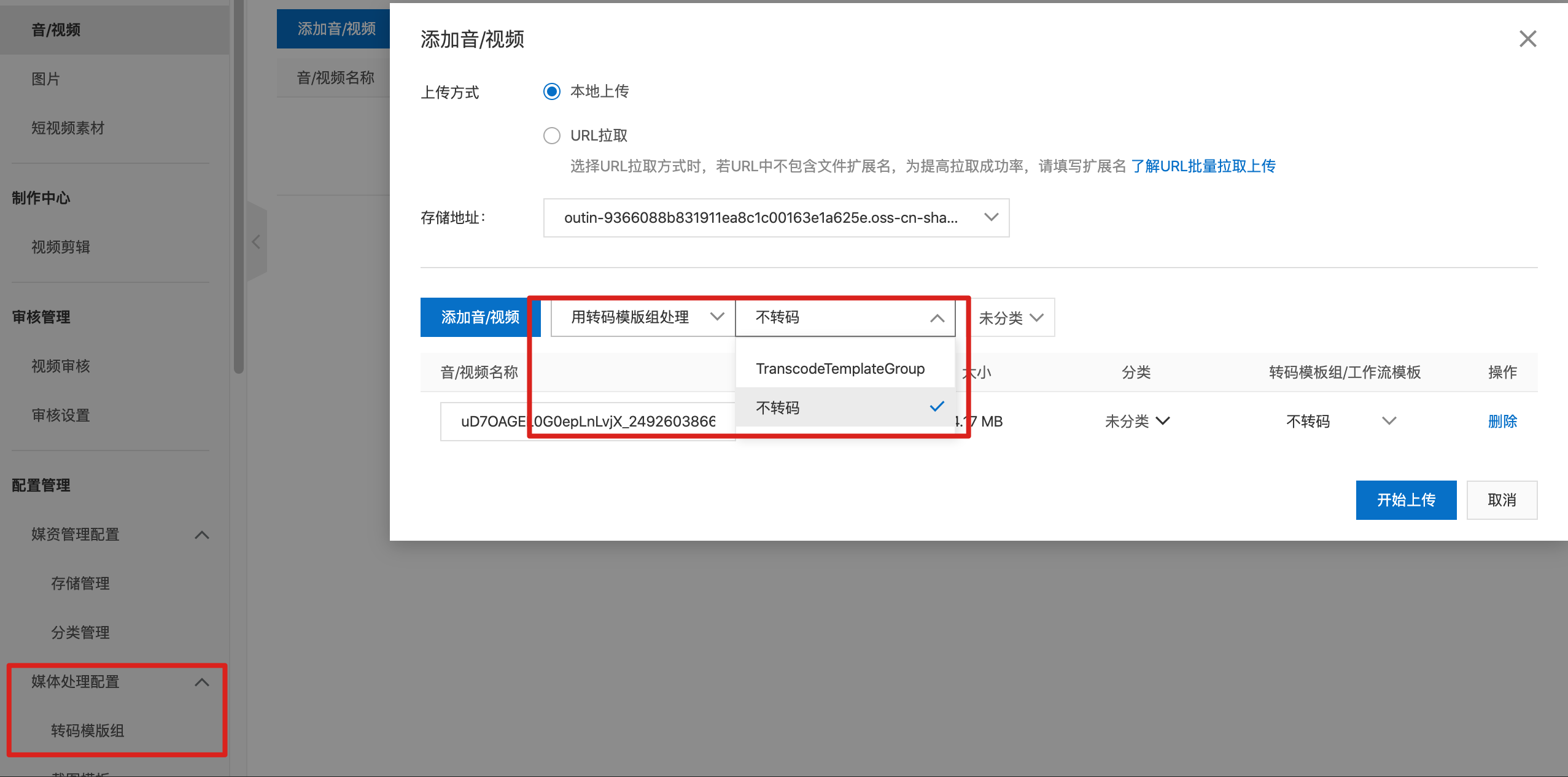
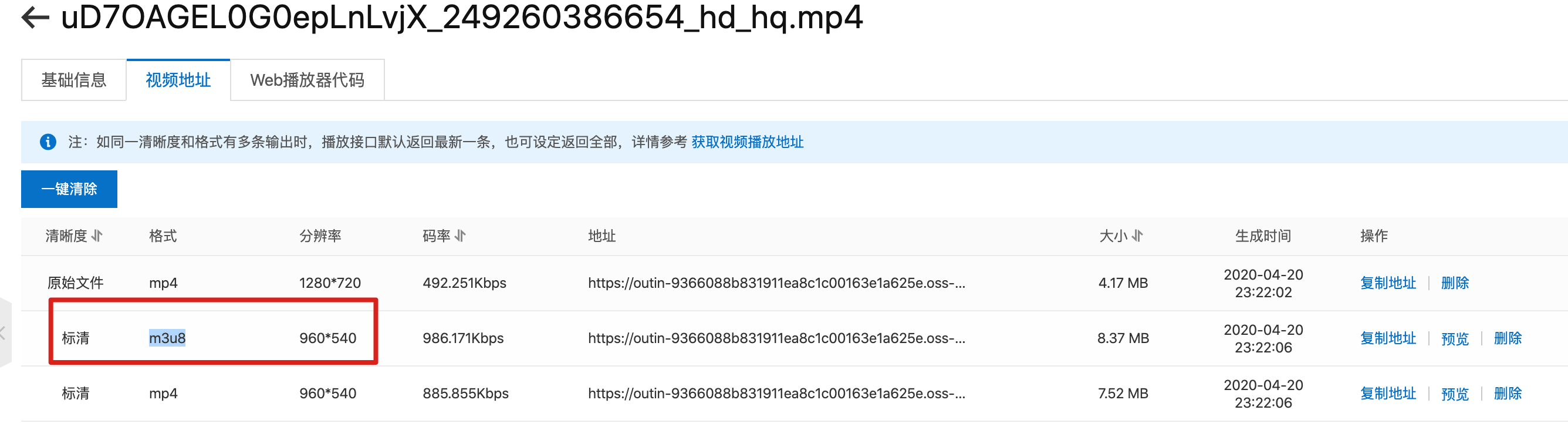
域名绑定
如果要加密视频的话一定要绑定域名并且域名备案。正在备案…TODO
账号设置
还是用我搭建OSS时创建的Access子账号,并在其所在用户组添加视频点播权限
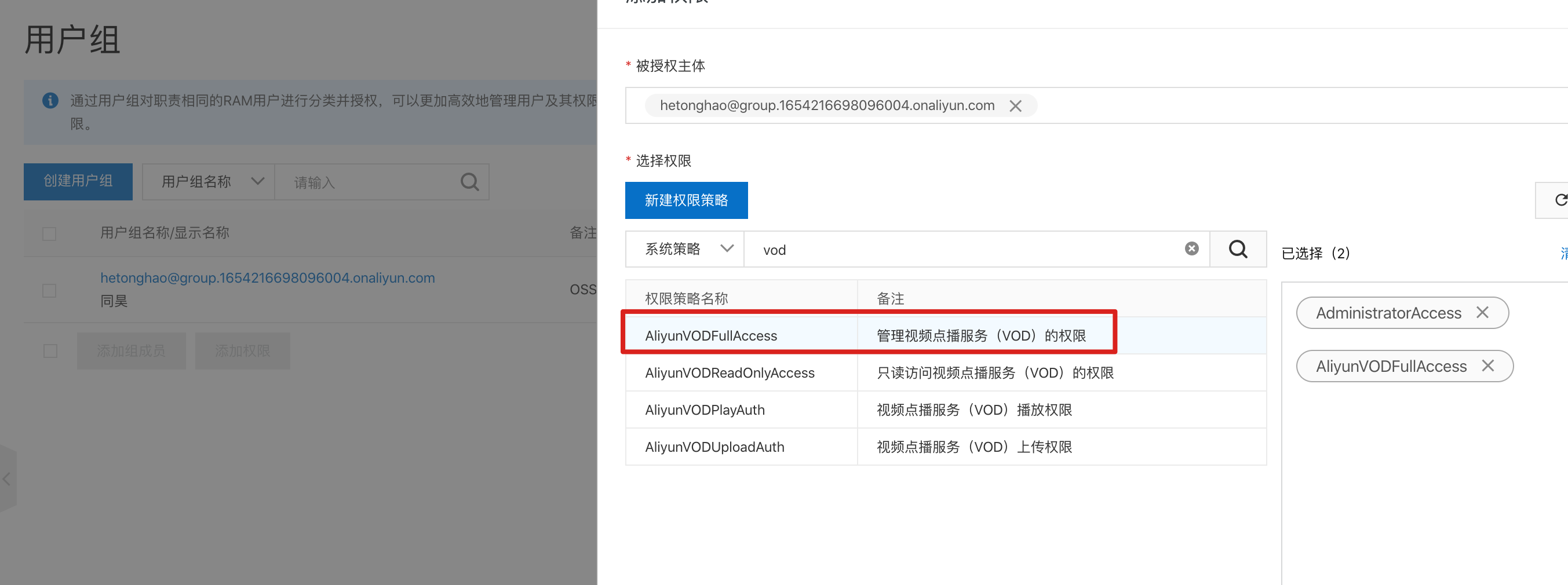
对接VoD SDK
参考官方Java SDK文档
https://help.aliyun.com/document_detail/57756.html?spm=a2c4g.11186623.6.906.e692192bBnfacC
Java对接代码
添加Maven仓库
<repositories> <repository> <id>sonatype-nexus-staging</id> <name>Sonatype Nexus Staging</name> <url>https://oss.sonatype.org/service/local/staging/deploy/maven2/</url> <releases> <enabled>true</enabled> </releases> <snapshots> <enabled>true</enabled> </snapshots> </repository> </repositories>
|
添加pom依赖
<dependency> <groupId>com.aliyun</groupId> <artifactId>aliyun-java-sdk-core</artifactId> <version>4.3.3</version> </dependency> <dependency> <groupId>com.aliyun.oss</groupId> <artifactId>aliyun-sdk-oss</artifactId> <version>3.1.0</version> </dependency> <dependency> <groupId>com.aliyun</groupId> <artifactId>aliyun-java-sdk-vod</artifactId> <version>2.15.2</version> </dependency> <dependency> <groupId>com.alibaba</groupId> <artifactId>fastjson</artifactId> <version>1.2.28</version> </dependency> <dependency> <groupId>org.json</groupId> <artifactId>json</artifactId> <version>20170516</version> </dependency> <dependency> <groupId>com.google.code.gson</groupId> <artifactId>gson</artifactId> <version>2.8.2</version> </dependency>
|
添加依赖的两种方式
直接添加到项目resourses/lib下
添加到Maven仓库
mvn install:install-file -DgroupId=组名 -DartifactId=依赖 -Dversion=版本 - Dpackaging=jar -Dfile=文件名
|

添加未开源的本地依赖
<dependency> <groupId>com.aliyun</groupId> <artifactId>aliyun-java-vod-upload</artifactId> <version>1.4.12</version> </dependency>
|
配置application.yml
server: port: 8092 spring: servlet: multipart: max-file-size: 100MB vod: aliyun: accessKeyId: LTAI4FgBBEeeRTMkVVFkKFEA accessKeySecret: -----------------------
|
VodConfigProperties
package cn.hetonghao.oss.config;
import lombok.Data; import org.springframework.boot.context.properties.ConfigurationProperties;
@Data @ConfigurationProperties(prefix = "vod.aliyun") public class VodConfigProperties {
private String accessKeyId; private String accessKeySecret; }
|
IVodService
package cn.hetonghao.oss.service;
import org.springframework.web.multipart.MultipartFile;
public interface IVodService {
String upload(MultipartFile multipartFile);
void removeVideo(String videoId); }
|
ServiceImpl
package cn.hetonghao.oss.service.impl;
import cn.hetonghao.oss.config.VodConfigProperties; import cn.hetonghao.oss.service.IVodService; import com.aliyun.vod.upload.impl.UploadVideoImpl; import com.aliyun.vod.upload.req.UploadStreamRequest; import com.aliyun.vod.upload.resp.UploadStreamResponse; import com.aliyuncs.DefaultAcsClient; import com.aliyuncs.exceptions.ClientException; import com.aliyuncs.profile.DefaultProfile; import com.aliyuncs.vod.model.v20170321.DeleteVideoRequest; import com.aliyuncs.vod.model.v20170321.DeleteVideoResponse; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.boot.context.properties.EnableConfigurationProperties; import org.springframework.stereotype.Service; import org.springframework.web.multipart.MultipartFile;
import java.io.IOException; import java.io.InputStream;
@Service @EnableConfigurationProperties(VodConfigProperties.class) public class VodServiceImpl implements IVodService { @Autowired private VodConfigProperties vodConfigProperties;
@Override public String upload(MultipartFile multipartFile) { InputStream inputStream; try { inputStream = multipartFile.getInputStream(); } catch (IOException e) { e.printStackTrace(); return null; } String fileName = multipartFile.getOriginalFilename(); if (fileName == null) { return null; } String title = fileName.substring(0, fileName.lastIndexOf(".")); UploadStreamRequest request = new UploadStreamRequest(vodConfigProperties.getAccessKeyId() , vodConfigProperties.getAccessKeySecret(), title, fileName, inputStream); UploadVideoImpl uploader = new UploadVideoImpl(); UploadStreamResponse response = uploader.uploadStream(request); return response.getVideoId(); }
@Override public void removeVideo(String videoId) { try { DefaultAcsClient defaultAcsClient = initVodClient(vodConfigProperties.getAccessKeyId(), vodConfigProperties.getAccessKeySecret()); DeleteVideoRequest request = new DeleteVideoRequest(); request.setVideoIds(videoId); DeleteVideoResponse response = defaultAcsClient.getAcsResponse(request); } catch (ClientException e) { e.printStackTrace(); } }
private static DefaultAcsClient initVodClient(String accessKeyId, String accessKeySecret) { String regionId = "cn-shanghai"; DefaultProfile profile = DefaultProfile.getProfile(regionId, accessKeyId, accessKeySecret); return new DefaultAcsClient(profile); } }
|
VideoController
@Api("vod视频点播") @RestController @RequestMapping("video") public class VideoController { @Autowired private IVodService vodService;
@ApiOperation("视频上传") @PostMapping("upload") public SingleResponse<String> upload(@RequestParam MultipartFile file) { return new SingleResponse<String>() .setData(vodService.upload(file)); }
@ApiOperation("删除视频") @DeleteMapping("{videoId}") public SingleResponse<String> upload(@PathVariable("videoId") String videoId) { vodService.removeVideo(videoId); return new SingleResponse<>(); } }
|
测试
测试上传视频
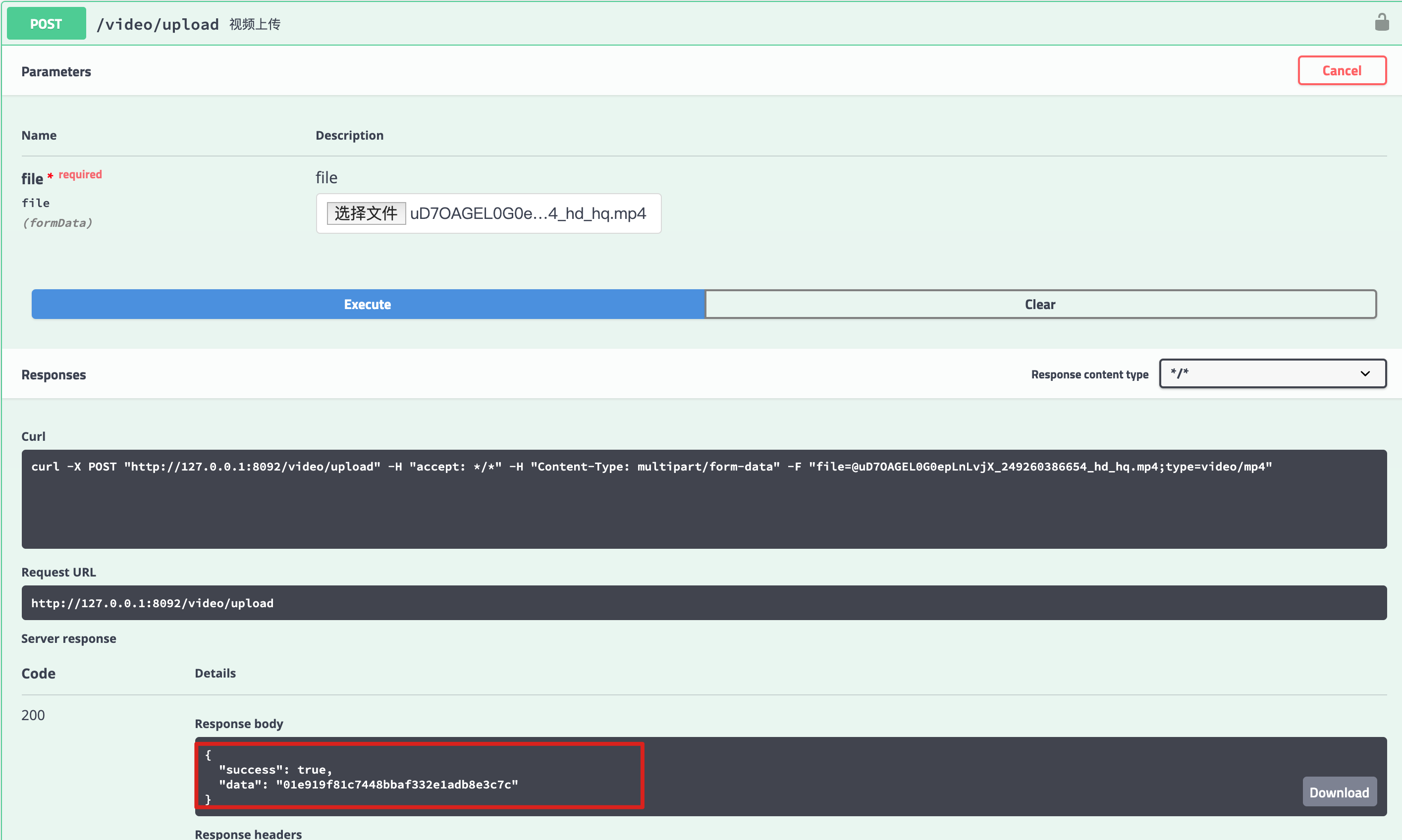
进入VoD后台查看

测试删除视频
集成视频播放器
Aliplayer提供了在线生成视频播放器的页面,可根据自己的需求生成相应的视频播放器代码:Aliplayer在线生成
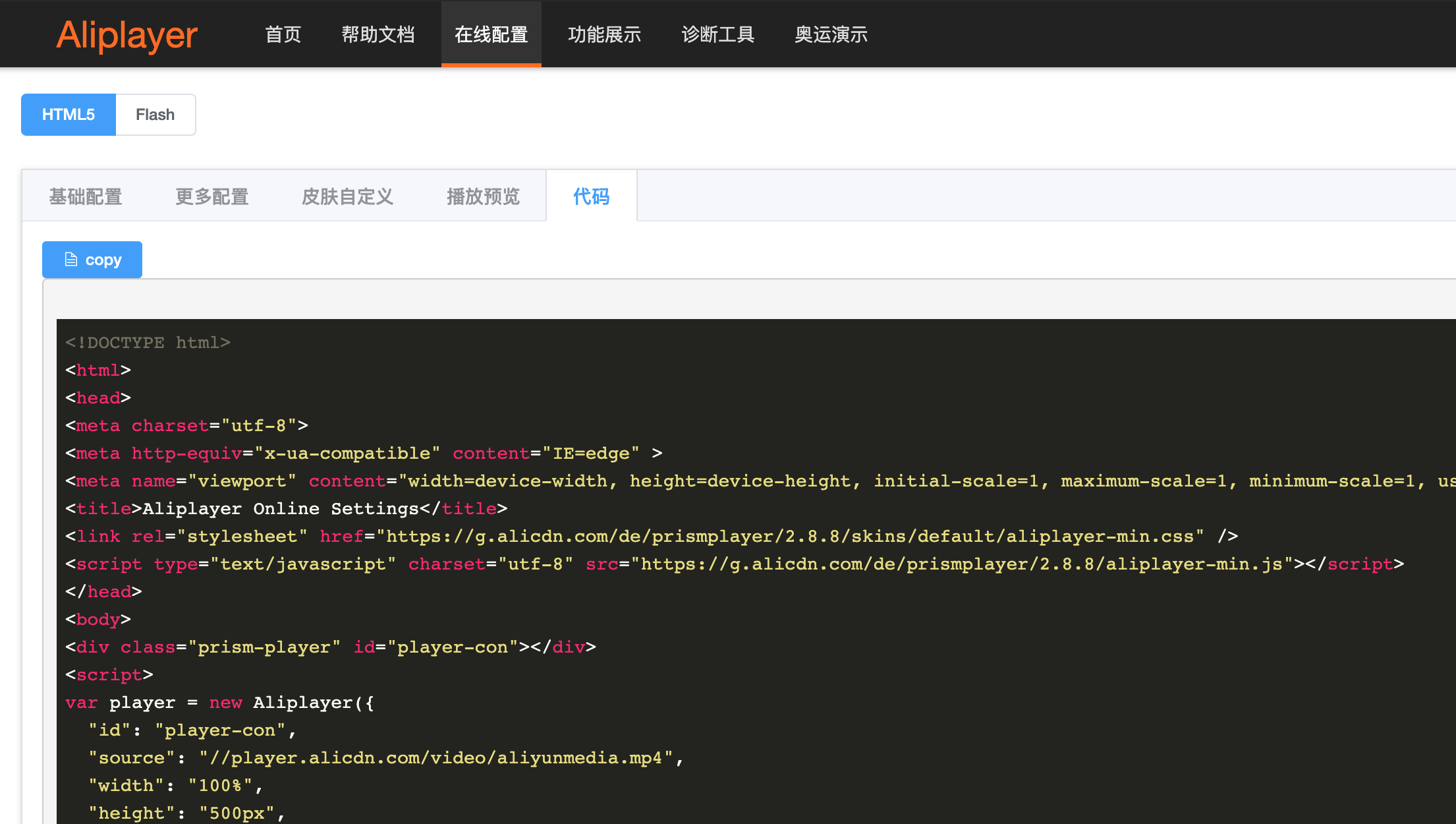